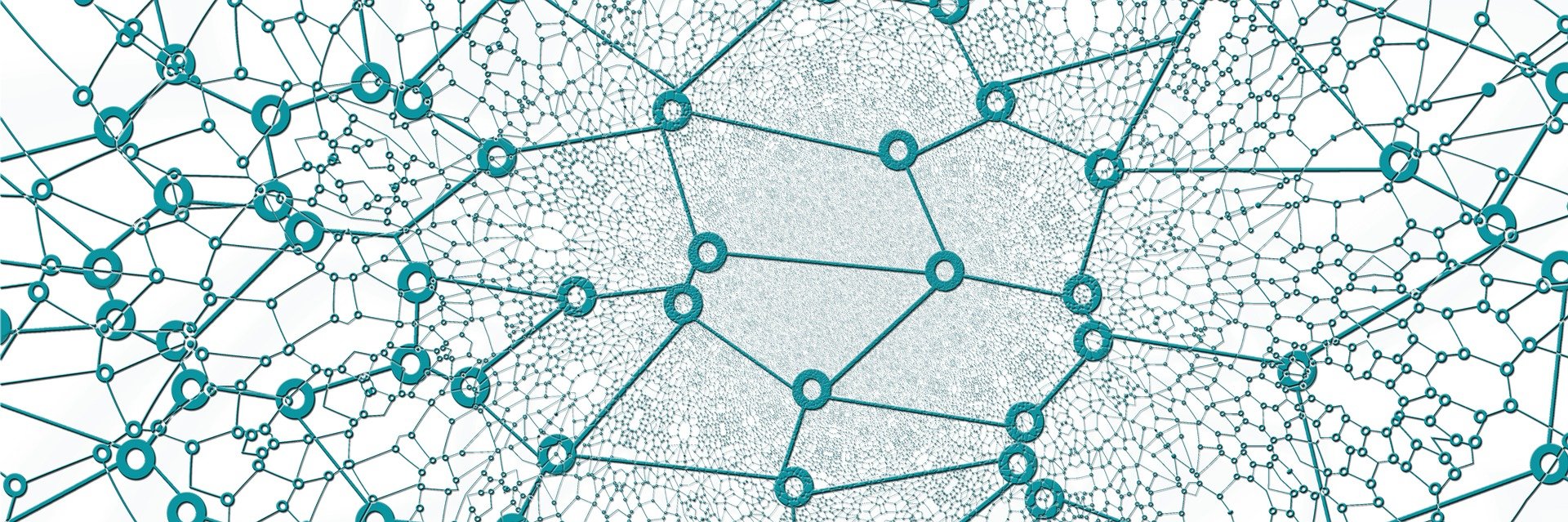
AsyncStorage
준비물
- react-native
- npm install –save @react-native-community/async-storage
Code
Part.import
import React from 'react';
import { View, Text, StyleSheet, TouchableOpacity } from 'react-native';
import AsyncStorage from '@react-native-community/async-storage';
Part.variable
Part.app
function App() {
setItemStorage = async (key, value) => {
try {
await AsyncStorage.setItem(key,value);
} catch (error) {
// Error save data
console.log("save data error")
}
};
removeItemStorage = async (key) => {
try {
await AsyncStorage.removeItem(key);
} catch (error) {
// Error remove data
console.log("remove data error")
}
};
getItemStorage = async (key) => {
try {
const value = await AsyncStorage.getItem(key);
if (value!==null) {
return value
} else {
console.log("read data error")
}
} catch (error) {
// Error read data
console.log("read data error")
}
};
saveStorage = () => {
this.setItemStorage("LOGIN", JSON.stringify({id:"id01", pw:"pw01"}))
}
readStorage = () => {
this.getItemStorage("LOGIN").then(result => {
const jsonObject = JSON.parse(result)
alert("username : "+jsonObject.id+" password : "+jsonObject.pw)
})
}
removeStorage = () => {
this.removeItemStorage("LOGIN")
}
const {container, btnStyle, txtStyle} = styles;
return (
<View style={container}>
<TouchableOpacity style={btnStyle} onPress={this.saveStorage}>
<Text style={txtStyle}>Save Data</Text>
</TouchableOpacity>
<TouchableOpacity style={btnStyle} onPress={this.readStorage}>
<Text style={txtStyle}>Read Data</Text>
</TouchableOpacity>
<TouchableOpacity style={btnStyle} onPress={this.removeStorage}>
<Text style={txtStyle}>Remove Data</Text>
</TouchableOpacity>
</View>
);
}
Part.style
const styles = StyleSheet.create({
container:{
flex:1,
justifyContent:'center',
alignItems:'center'
},
btnStyle:{
backgroundColor:'#7f7fff',
justifyContent:'center',
height:50,
width:100,
marginTop:10,
alignItems:'center'
},
txtStyle:{
color:'#fff'
}
})
TIL
JSON
JSON.stringify({id:"id01", pw:"pw01"})
const jsonObject = JSON.parse(result)
- JSON 형식으로 Read/Write 할 때 JSON.stringify/JSON.parse가 사용된다.
AsyncStorage
import AsyncStorage from '@react-native-community/async-storage';
- Android의 경우 react-native에 기본 탑재된 async-storage를 사용할 수 있지만 ios의 경우 @react-native-community/async-storage를 설치해야 하는 것 같다.
- 용도는 알다시피 LocalStorage처럼 사용된다.